Bypassing GPT-4's Context Length Limitation with Sliding Window Technique
Brian Baliach
- 19 May 2023
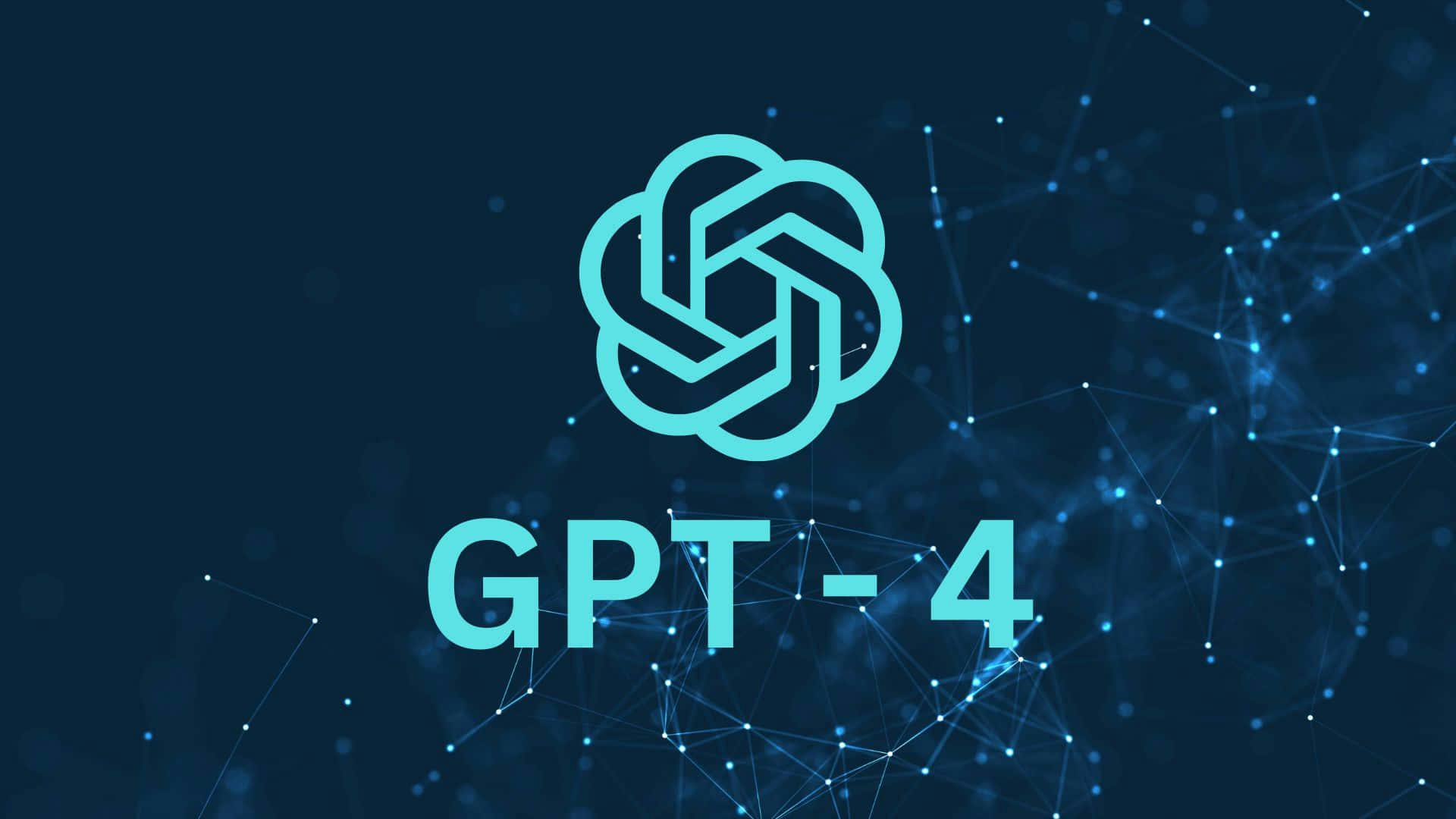
GPT-4, despite its incredible linguistic prowess, suffers from a noteworthy constraint: its context length limitation, which essentially refers to the maximum number of input tokens. The sliding window technique, however, serves as a workaround by breaking up a document into overlapping segments that GPT-4 can easily digest.
In this blog post, we'll examine the implementation of the sliding window technique to bypass GPT-4's context length restriction, discuss associated trade-offs, and identify optimal chunk and overlap lengths for best results.
Harnessing the Sliding Window Technique
The sliding window technique entails dividing a document into smaller segments within GPT-4's context length limit, processing each segment, and then reassembling them. The primary challenge when employing this method is preserving context and fluidity among processed segments.
To accomplish this, we establish a chunk length and an overlap length that guarantee context continuity. The chunk length must be equal to or less than GPT-4's context length limit, while the overlap length should be adequately large to facilitate contextual comprehension between processed segments.
For instance, if GPT-4's context length limit is 2048 tokens, we might use a chunk length of 2000 tokens and an overlap length of 100 tokens. This way, when stitching processed segments back together, the overlapping sections help maintain context and minimize duplication.
Here's a TypeScript example illustrating how to implement this. Note that the gpt4Function
should be your helper function responsible for making API calls to OpenAI.
async function processTextFile(filePath: string, chunkLength: number, overlapLength: number): Promise<void> {
const readInterface = readline.createInterface({
input: fs.createReadStream(filePath),
output: process.stdout,
terminal: false
});
let buffer = '';
let result = '';
let isFirstChunk = true;
const outputFile = filePath.replace('.txt', '_new.txt');
for await (const line of readInterface) {
buffer += ' ' + line;
while (buffer.length >= chunkLength) {
let chunk = buffer.slice(0, chunkLength);
buffer = buffer.slice(chunkLength - overlapLength);
let processedChunk = await gpt4Function(chunk);
if (isFirstChunk) {
result += processedChunk;
isFirstChunk = false;
} else {
result += processedChunk.slice(overlapLength);
}
}
fs.writeFileSync(outputFile, result, {flag: 'w'});
}
if (buffer.length > 0) {
let lastProcessedChunk = await gpt4Function(buffer);
result += lastProcessedChunk.slice(overlapLength);
}
fs.writeFileSync(outputFile, result, {flag: 'w'});
}
Selecting the Ideal Chunk Length and Overlap Length
Striking the perfect balance between chunk length and overlap length is key to achieving optimal results. Begin by setting the chunk length close to GPT-4's context length limit and determining a reasonable overlap length based on empirical testing (trial and error).
Applications
- Summarizing Large Documents: The sliding window technique makes summarizing extensive articles much more manageable, delivering coherent and precise summaries to users.
- Proofreading and Grammar Correction: Massive text files can be swiftly checked for grammar mistakes and punctuation problems.
- Plagiarism Detection: By utilizing the sliding window technique to process large volumes of text, plagiarism in academic or corporate environments can be detected.
Limitations
While the sliding window technique is undeniably useful for processing large documents and generating consistent and accurate input, it does come with certain drawbacks. For example, when summarizing large documents, small overlaps may be insufficient for preserving context in specific cases, potentially resulting in a loss of meaning or continuity. Furthermore, this method increases usage costs. OpenAI charges per API call, so making multiple calls for different chunks will drive up costs.
Conclusion
Although we continue to grapple with GPT-4's context length limitation, the sliding window technique allows us to exploit GPT-4's full potential across a range of applications such as summarization, proofreading, and plagiarism detection. The next GPT-4 iteration will feature a much larger context window which will help circumvent some limitations of using this technique.